Often on the GEF newsgroup there can be found questions concerning zooming in the GEF editor. I tried to investigate this subject and found out that adding basic zooming operations isn't to complicated. All required code I found in the Logic GEF example project: org.eclipse.gef.examples.logic project, which can be checkout from the CVS (if you don't know how to access Eclipse CVS check this page: WIKI CVS HowTo).
To demonstrate the solution I will use another GEF example, but this time it will be Shape Editor project: org.eclipse.gef.examples.shapes, which also can be checkout form the CVS. Shape Editor is very simple GEF editor, which allows you only to add two figures and connect them with two connection types. We will want to add the zooming facility to this poor functionality.
Zoom actions can be added in two ways:
- by the toolbar
- by the menu
- In the class
org.eclipse.gef.examples.shapes.ShapesEditorActionBarContributor you need to overwrite method
EditorActionBarContributor#contributeToToolBar
and add this code:
String[] zoomStrings = new String[] {
ZoomManager.FIT_ALL, ZoomManager.FIT_HEIGHT,
ZoomManager.FIT_WIDTH
};
toolBarManager.add(newZoomComboContributionItem(getPage(), zoomStrings));
- in the class org.eclipse.gef.examples.shapes.ShapesEditor
in the method configureGraphicalViewer
you need to add such code:
List zoomLevels = new ArrayList(3);
zoomLevels.add(ZoomManager.FIT_ALL);
zoomLevels.add(ZoomManager.FIT_WIDTH);
zoomLevels.add(ZoomManager.FIT_HEIGHT);
root.getZoomManager().setZoomLevelContributions(zoomLevels);
- in the class org.eclipse.gef.examples.shapes.ShapesEditor
in the method getAdapter
you need to add such code:
if (type == ZoomManager.class) {
return getGraphicalViewer().getProperty(ZoomManager.class.toString());
}
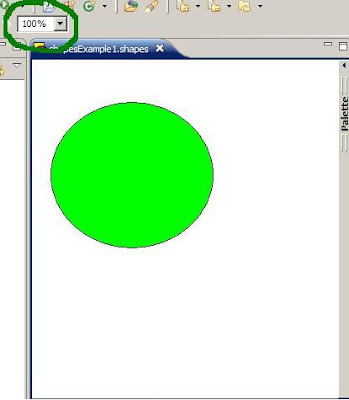
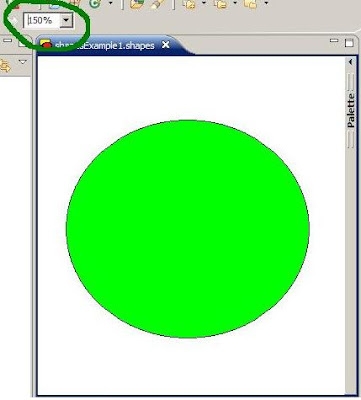
Now let's see what are we suppose to do to add the zooming to the main Eclipse menu
- in the class org.eclipse.gef.examples.shapes.ShapesEditor in the method configureGraphicalViewer you need to add such code:
IAction zoomIn = new ZoomInAction(root.getZoomManager());
IAction zoomOut = new ZoomOutAction(root.getZoomManager())
getActionRegistry().registerAction(zoomIn);
getActionRegistry().registerAction(zoomOut);
and additionaly if you want to have the keyboard shortcuts you have to add:
getSite().getKeyBindingService().registerAction(zoomIn);
getSite().getKeyBindingService().registerAction(zoomOut);
- in the class org.eclipse.gef.examples.shapes.ShapesEditorActionBarContributoryou need to overwrite method
EditorActionBarContributor#contributeToMenu(IMenuManager)and add there:
super.contributeToMenu(menubar);
MenuManager viewMenu = newMenuManager("ZOOM");
viewMenu.add(getAction(GEFActionConstants.ZOOM_IN));
viewMenu.add(getAction(GEFActionConstants.ZOOM_OUT));
menubar.insertAfter(IWorkbenchActionConstants.M_EDIT,viewMenu); //where the menu should placed on the menu bar
The effect you can see below:
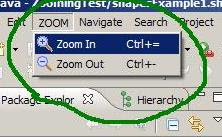
4 comments:
Hi,
Nice concise tutorial. Helped me a lot !!
Thanks.
Regards,
Jeet
Hello.
I would like what does menubar mean in the last portion of code.
Thanks.
Hi,
nice tutorial!
Do you have an idea to use the Drop Down Menu to select the zoom level with a Multipage Editor? Because the ZoomComboContributionItem gets connected with only one viewer.
Regards,
Timo
hello
For me menu bar Zoom in Zoom Out not working.....
i can see all the content appearing on the Menu.....i can able to click on that Menu.... But i cant see any effect on my Editor.....
regards,
Karthikeyan
Post a Comment